2 min to read
Overview of Static Factory Methods
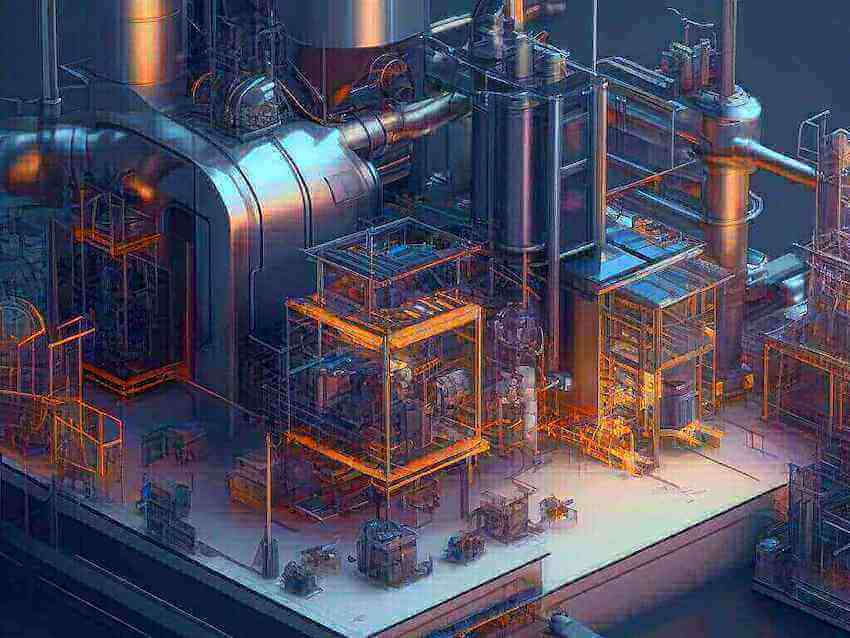
Short summary of Effective Java 3rd edition book - Chapter 2
Static factory method is not factory method. They are two different things.
Descriptive Method Names
Static factory methods can have names that describe the purpose of the method, making the code more readable and self-explanatory.
public static BigDecimal valueOf(long b){...}
in the case of simple objects, it is difficult to see the benefit of using static factory methods but for me, it is more natural to use valueOf than constructor
BigDecimal.valueOf(1)
instead of constructornew BigDecimal(1)
much more sense we can see when object is a bit more complicated like:
Duration.ofSeconds(1)
orDuration.ofDays(1)
This method is way more readable than the constructor and in this particular case, it is not even possible
to new Duration(...)
because contructor is private
No need to create new instance each time
Static methods are associated with the class itself
, not with any specific object instance
.
As a result, static methods can be called on the class without creating an instance of the class.
This can be advantageous and disadvantageous at the same time.
public class Example {
private static int count = 0;
// Static method
public static void incrementCount() {
count++;
}
// Instance method
public void displayCount() {
System.out.println("Count: " + count);
}
public static void main(String[] args) {
// Calling static method without creating an instance
Example.incrementCount(); // Count is now 1
// Creating instances
Example obj1 = new Example();
Example obj2 = new Example();
// Calling instance method on obj1
obj1.displayCount(); // Displays Count: 1
// Calling static method again
Example.incrementCount(); // Count is now 2
// Calling instance method on obj2
obj2.displayCount(); // Displays Count: 2
}
}
Caching
We can implement caching mechanisms, which can be useful when creating immutable objects or when there is a need to reuse previously created instances.
private static Map<String, MyClass> cache=new HashMap<>();
public static MyClass getInstance(String key){
if(!cache.containsKey(key)){
cache.put(key,new MyClass(key));
}
return cache.get(key);
}
Comments